Write to Blockchain
Sign Message
Sign a given message as a hex string. For example, you can use this signature to validate content on your backend.
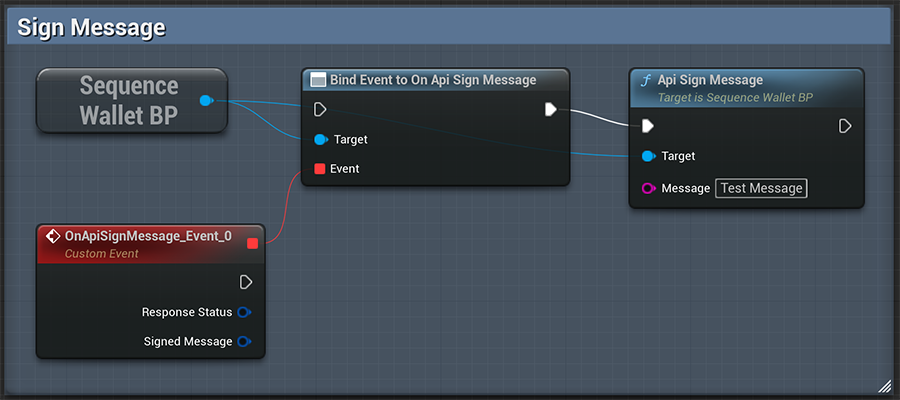
const TSuccessCallback<FSeqSignMessageResponse_Response> OnResponse = [this] (const FSeqSignMessageResponse_Response& Response)
{
//Response is the signed message
};
const FFailureCallback OnFailure = [this](const FSequenceError& Error)
{
UE_LOG(LogTemp,Display,TEXT("Error Message: %s"),*Error.Message);
};
const TOptional<USequenceWallet*> WalletOptional = USequenceWallet::Get();
if (WalletOptional.IsSet() && WalletOptional.GetValue())
{
USequenceWallet* Wallet = WalletOptional.GetValue();
const FString Message = "Hi";
Wallet->SignMessage(Message, OnResponse, OnFailure);
}
Send Native Tokens
Send native token balances to other users, such as sending ETH on the Ethereum Mainnet.
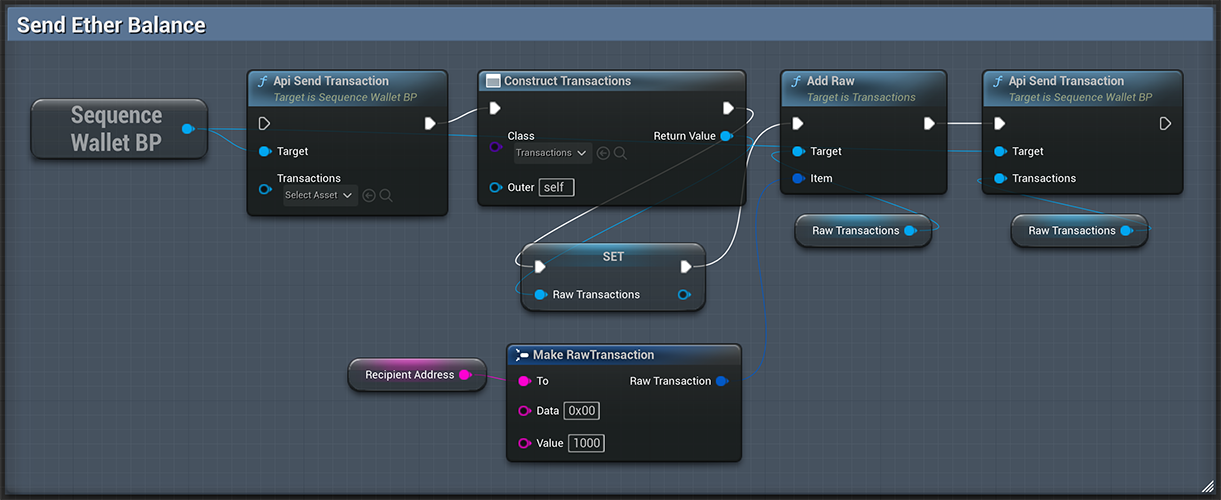
FRawTransaction T;
T.data = "0x0";
T.to = ToAddress;
T.value = "1";
// Append the Raw Transaction
Txn.Push(TUnion<FRawTransaction, FERC20Transaction, FERC721Transaction, FERC1155Transaction, FDelayedTransaction>(T));
Send ERC20 Tokens
Send your custom ERC20 tokens from Builder to users, or transfer existing tokens like USDC or WETH.
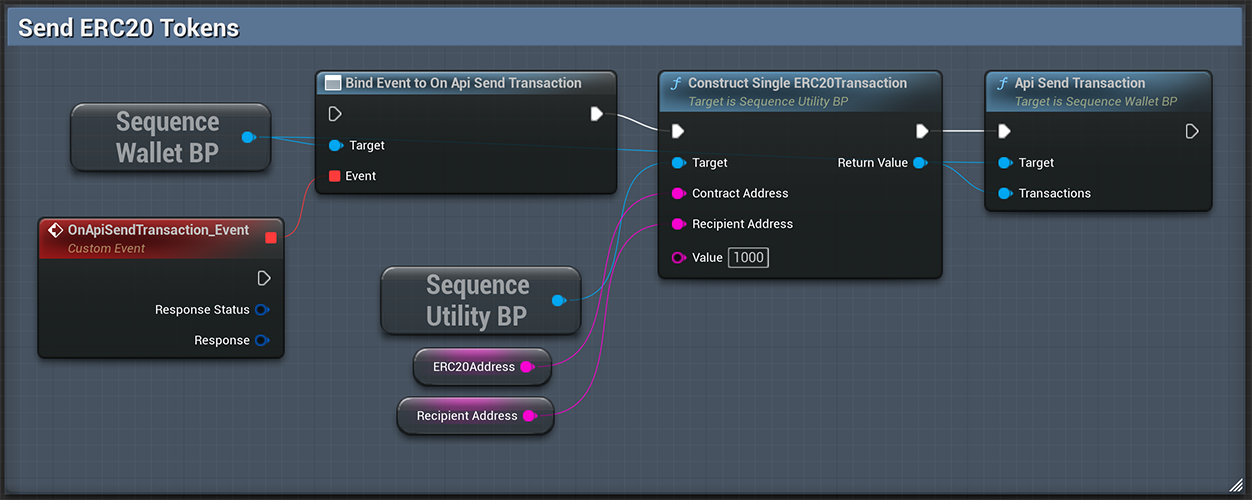
// Create the ERC20 transaction
FERC20Transaction T20;
T20.to = "0x0E0f9d1c4BeF9f0B8a2D9D4c09529F260C7758A2";
T20.tokenAddress = "0x2791Bca1f2de4661ED88A30C99A7a9449Aa84174";
T20.value = "1000";
// Append the ERC20 transaction to the Txn object
Txn.Push(TUnion<FRawTransaction, FERC20Transaction, FERC721Transaction, FERC1155Transaction, FDelayedTransaction>(T20));
Send NFTs (ERC721 Tokens)
Enable your users to send NFTs to others.
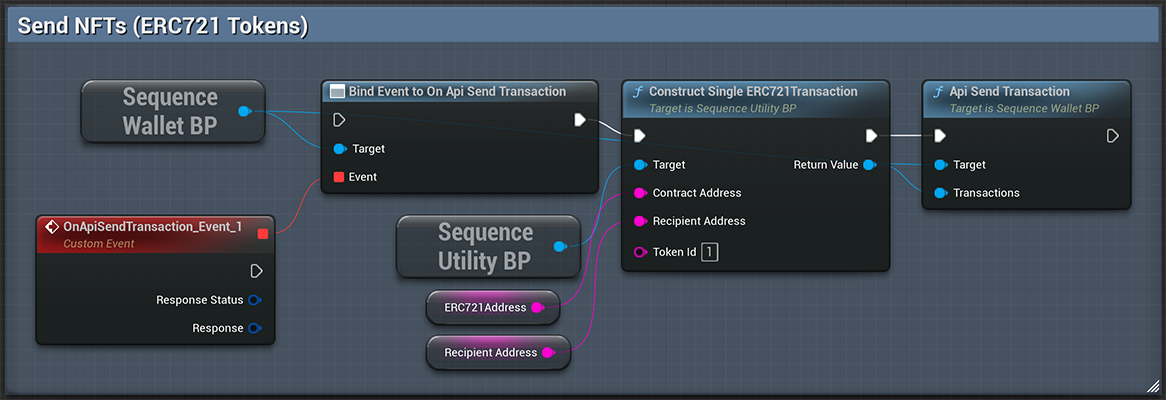
// Create the ERC721 transaction
FERC721Transaction T721;
T721.safe = true;
T721.id = "54530968763798660137294927684252503703134533114052628080002308208148824588621";
T721.to = "0x0E0f9d1c4BeF9f0B8a2D9D4c09529F260C7758A2";
T721.tokenAddress = "0xa9a6A3626993D487d2Dbda3173cf58cA1a9D9e9f";
// Append the ERC721 transaction to the Txn object
Txn.Push(TUnion<FRawTransaction, FERC20Transaction, FERC721Transaction, FERC1155Transaction, FDelayedTransaction>(T721));
Send Collectibles (ERC1155 Tokens)
Enable your users to send Collectibles to others.
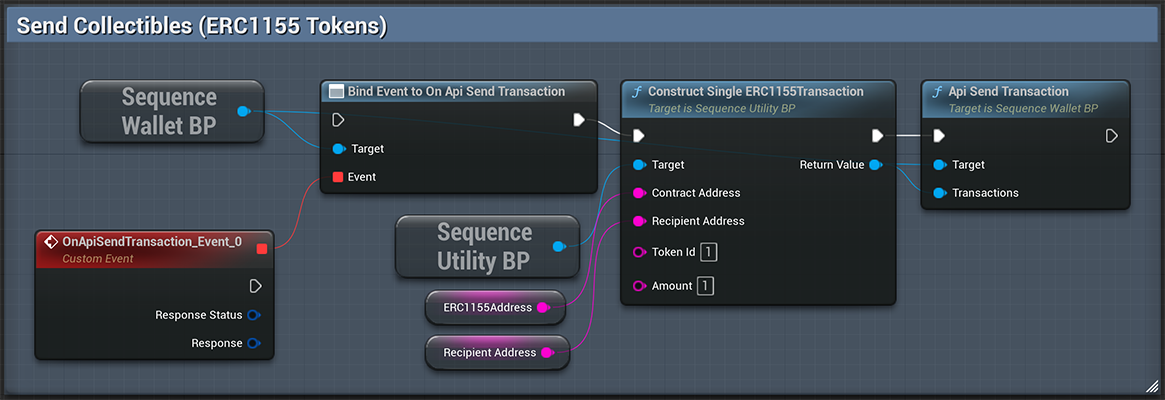
// Create the ERC1155 transaction
FERC1155Transaction T1155;
T1155.to = "0x0E0f9d1c4BeF9f0B8a2D9D4c09529F260C7758A2";
T1155.tokenAddress = "0x631998e91476DA5B870D741192fc5Cbc55F5a52E";
FERC1155TxnValue Val;
Val.amount = "1";
Val.id = "66635";
T1155.vals.Add(Val);
// Append the ERC1155 transaction to the Txn object
Txn.Push(TUnion<FRawTransaction, FERC20Transaction, FERC721Transaction, FERC1155Transaction, FDelayedTransaction>(T1155));
Send Raw Transactions
Note: if you want call contracts with the Raw type you'll want include the header #include "ABI/ABI.h" in order to use the ABI to encode the data for a contract call.
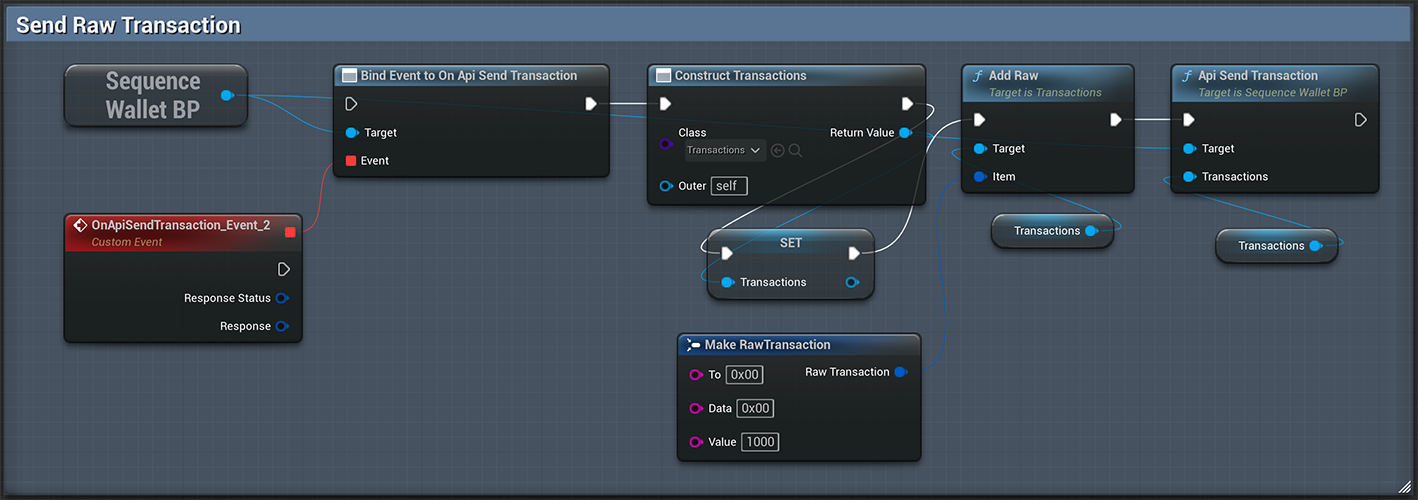
void UMyClass::BurnToken(TArray<TUnion<FRawTransaction, FERC20Transaction, FERC721Transaction, FERC1155Transaction, FDelayedTransaction>> Txn, FString ContractAddress, int32 TokenId, int32 Amount)
{
FString FunctionSignature = "burn(uint256,uint256)";
TFixedABIData ABITokenId = ABI::Int32(TokenId);
TFixedABIData ABIFixedAmount = ABI::Int32(Amount);
TArray<ABIEncodeable*> Arr;
Arr.Add(&ABITokenId);
Arr.Add(&ABIFixedAmount);
FUnsizedData EncodedData = ABI::Encode(FunctionSignature, Arr);
FRawTransaction T;
T.data = "0x" + EncodedData.ToHex();
T.to = ContractAddress;
T.value = "0"
// Append the Raw Transaction
Txn.Push(TUnion<FRawTransaction, FERC20Transaction, FERC721Transaction, FERC1155Transaction, FDelayedTransaction>(T));
}
Send Delayed Encode Transactions
When working with Blueprints, it is easier to call a contract via server-side encoding using a Delayed Encode transaction.
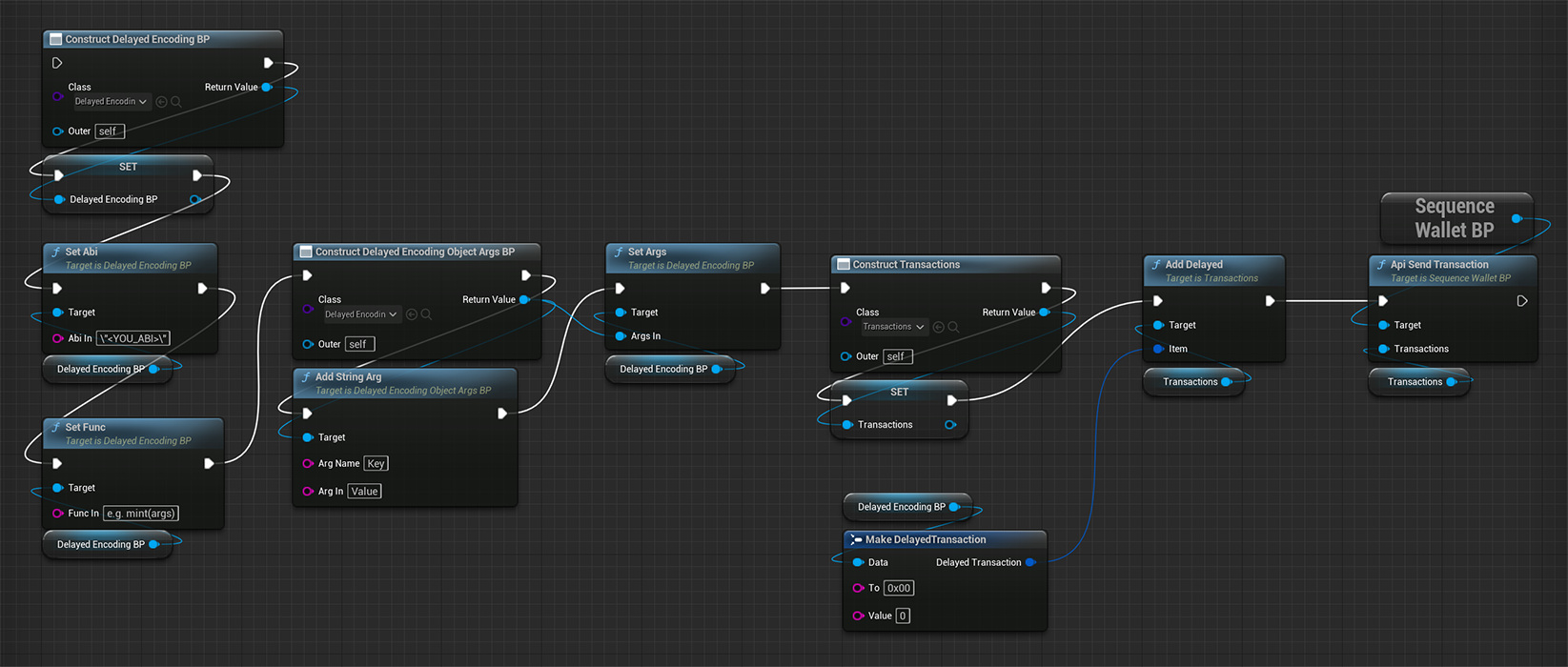
FDelayedTransaction DelayedEncodeTxn;
DelayedEncodeTxn.to = ERC20Address;
DelayedEncodeTxn.value = TEXT("0");
UDelayedEncodingBP* data = NewObject<UDelayedEncodingBP>();
data->SetAbi(TEXT("mint(address,uint256)"));
UDelayedEncodingArrayArgsBP* args = NewObject<UDelayedEncodingArrayArgsBP>();
args->AddStringArg(WalletAddress);
args->AddStringArg("1");
data->SetArgs(args);
data->SetFunc(TEXT("mint"));
DelayedEncodeTxn.data = data;
Transactions.Add(TransactionUnion(DelayedEncodeTxn));
Send Transactions with Fee Options
To send transactions with fee options, first retrieve the available fee options using the GetFeeOptions
method.
Once the fee options are received, select a fee option and use the SendTransactionWithFeeOption
method to send
the transaction with the selected fee.
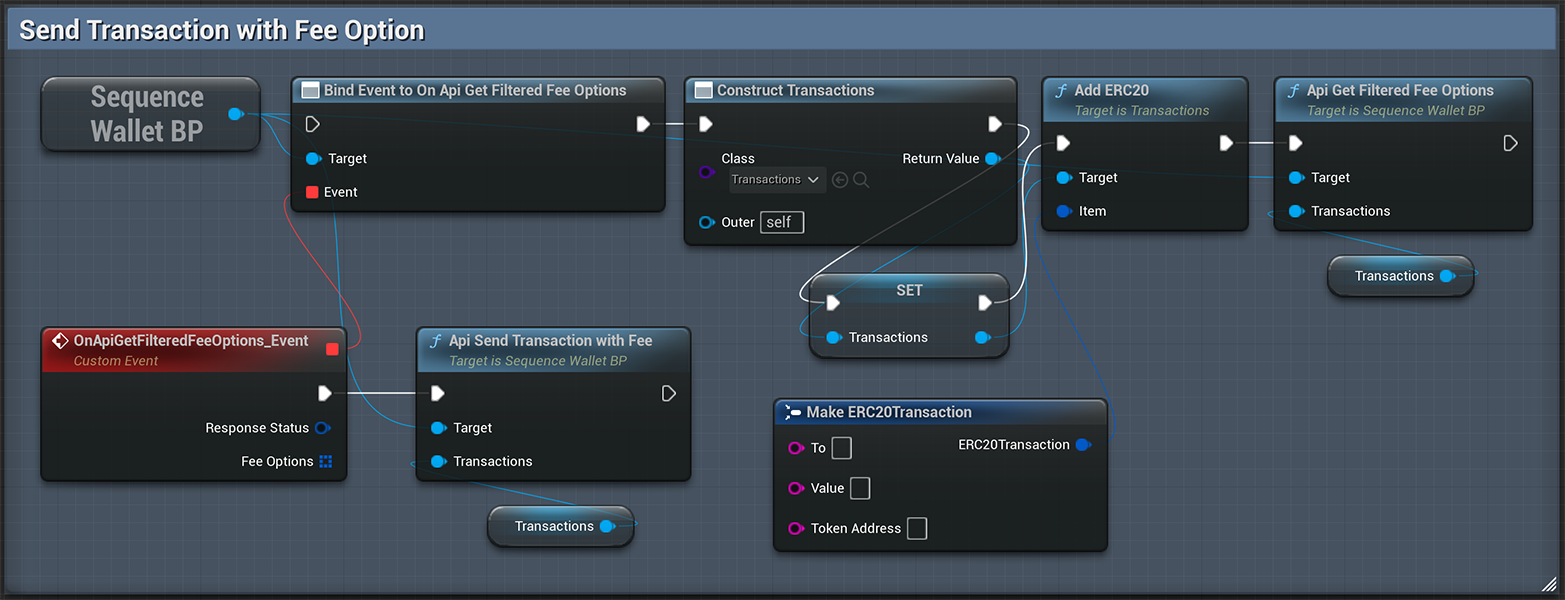
// Define the callback for handling the fee options response
const TFunction<void(TArray<FFeeOption>)> OnFeeResponse = [Transactions, OnSuccess, OnFailure](const TArray<FFeeOption>& Response)
{
if (Response.Num() > 0)
{
const FFeeOption SelectedFeeOption = Response[0];
UE_LOG(LogTemp, Display, TEXT("Using FeeOption: %s"), *UIndexerSupport::StructToString(SelectedFeeOption));
const FFailureCallback OnTransactionFailure = [OnFailure](const FSequenceError& Error)
{
OnFailure("Transaction failure", Error);
};
const UAuthenticator* Auth = NewObject<UAuthenticator>();
const TOptional<USequenceWallet*> WalletOptional = USequenceWallet::Get(Auth->GetStoredCredentials().GetCredentials());
if (WalletOptional.IsSet() && WalletOptional.GetValue())
{
USequenceWallet* Wallet = WalletOptional.GetValue();
Wallet->SendTransactionWithFeeOption(Transactions, SelectedFeeOption, [=](const FSeqTransactionResponse_Data& Transaction)
{
FString OutputString;
const TSharedRef<TJsonWriter<>> Writer = TJsonWriterFactory<>::Create(&OutputString);
FJsonSerializer::Serialize(Transaction.Json.ToSharedRef(), Writer);
OnSuccess(OutputString);
}, OnTransactionFailure);
}
}
else
{
OnFailure("Test failed no fee options in response", FSequenceError(EErrorType::EmptyResponse, "Empty fee option response"));
}
};
// Define the callback for handling fee options retrieval failure
const FFailureCallback OnFeeFailure = [OnFailure](const FSequenceError& Error)
{
OnFailure("Get Fee Option Response failure", Error);
};
// Retrieve fee options and send the transaction with the selected fee option
const UAuthenticator* Auth = NewObject<UAuthenticator>();
const TOptional<USequenceWallet*> WalletOptional = USequenceWallet::Get(Auth->GetStoredCredentials().GetCredentials());
if (WalletOptional.IsSet() && WalletOptional.GetValue())
{
USequenceWallet* Wallet = WalletOptional.GetValue();
Wallet->GetFeeOptions(Transactions, OnFeeResponse, OnFeeFailure);
}