Getting Started with Sequence Kit
To spin up a quick boilerplate that leverages Sequence Kit, you can run the following command from your terminal to install the Sequence-CLI and create a react app:
npx sequence-cli boilerplates create-embedded-wallet-react-starter
Otherwise, we will walk you through the process of installing Sequence Kit, instantiating the connection modal, and displaying the Inventory in your application.
Installing Sequence Kit Packages
Sequence Kit is modular, allowing you to install only the necessary packages. To get started, install the @0xsequence/kit
core package, as well as install other dependencies such as wagmi
, viem
, and 0xsequence
.
npm install @0xsequence/kit @0xsequence/waas wagmi [email protected] viem 0xsequence @0xsequence/design-system framer-motion @tanstack/react-query
# or
pnpm install @0xsequence/kit @0xsequence/waas wagmi [email protected] viem 0xsequence @0xsequence/design-system framer-motion @tanstack/react-query
# or
yarn add @0xsequence/kit @0xsequence/waas wagmi [email protected] viem 0xsequence @0xsequence/design-system framer-motion @tanstack/react-query
Setting Up your Dapp
To utilize the core kit wrapper for connecting web3 wallets to your application, follow these steps:
Create a React Project with Vite
Create a Config
Next, a configuration variable for Sequence Kit will need to be created as either a waas
(meaning an Embedded Wallet) or universal
(meaning a Universal Wallet) wallet type.
For waas
, first obtain a WaaS Config Key from the Sequence Builder, Wallet Connect ID, and setup other Login Provider configuration. For both wallet type options, obtain and use a project access key.
import { createConfig } from '@0xsequence/kit'
export const config: any = createConfig('waas' /*or, 'universal'*/, {
projectAccessKey: '<your-project-access-key>',
chainIds: [1, 137],
defaultChainId: 1,
appName: 'Demo Dapp',
waasConfigKey: '<your-waas-config-key>', // for waas
google: {
clientId: '<your-google-client-id>'
},
walletConnect: {
projectId: '<your-wallet-connect-project-id>'
}
})
In order to customize further, you can view additional configuration parameters.
Setup Provider Component
The configuration we created in step 2 needs to be passed into the providers below in the main.tsx
, as well as the inclusion of the Sequence Design System styles.css
stylesheet:
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App'
import '@0xsequence/design-system/styles.css'
import { config } from './config'
function Dapp() {
return (
<SequenceKit config={config}>
<App/>
</SequenceKit>
);
}
ReactDOM.createRoot(document.getElementById('root')!).render(
<React.StrictMode>
<Dapp />
</React.StrictMode>,
)
For web3 interactions, wagmi exposes a set of React hooks that make it convenient for common functions like sending transactions. Please check out our Embedded Wallet boilerplate with Sequence Kit for more examples of interactions.
Toggling the Connect Modal
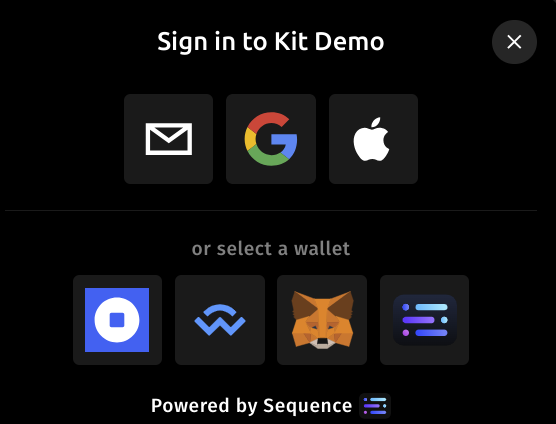
Invoke the connect modal using the useOpenConnectModal
hook.
import { useOpenConnectModal } from '@0xsequence/kit'
import { useDisconnect, useAccount } from 'wagmi'
export const App = () => {
const { setOpenConnectModal } = useOpenConnectModal()
const { isConnected } = useAccount()
const { disconnect } = useDisconnect()
return (
<>
{!isConnected && (
<button onClick={() => setOpenConnectModal(true)}>
Sign in
</button>
)}
{isConnected && (
<button onClick={() => disconnect()}>
sign out
</button>
)}
</>
)
}
The modal will automatically close once the user signs in. You can utilize the useAccount
hook from wagmi to detect the user's connection status.
Setting Up Inventory
To add the optional Inventory feature, add the KitWalletProvider
below the SequenceKit
wrapper.
import { KitWalletProvider } from '@0xsequence/kit-wallet'
// ...
const Dapp = () => {
return (
<SequenceKit config={config}>
<KitWalletProvider> // <-- Added code
<App />
</KitWalletProvider>
</SequenceKit>
)
}
Invoking the Inventory modal
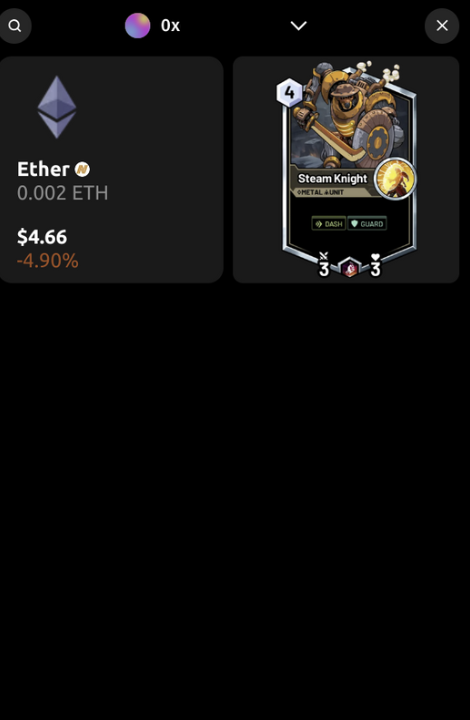
Open the Inventory using the useOpenWalletModal
react hook.
import { useOpenWalletModal } from '@0xsequence/kit-wallet'
const MyComponent = () => {
const { setOpenWalletModal } = useOpenWalletModal()
return (
<button onClick={() => setOpenWalletModal(true)}>open inventory</button>
)
}
Users can then select on the collectible in order to send to another address.

React Example
The Sequence Kit Github repository contains an example app that you can use for learning and testing.